Creating small executables with Qt Creator and MingW
Starting with an empty Plain C Project in Qt Creator IDE and gcc from MinGW as compiler, I will show you how to generate small binaries that are independent from MinGW dlls. At the writing of this article the app versions that I used were Qt Creator 2.6.2 with Qt 5.0.1 and gcc 4.7.2.
Replace the code of the main.c with following:
#include <windows.h>
void main()
{
MessageBoxA(0, "Hello", "world!", MB_OK);
ExitProcess(0);
}
Switch the build configuration in menu Projects from Debug to Release and compile the project (Ctrl + B is the default shortcut for this). This will result in a 9.728 byte big exe file. The file content, split in parts, looks like this:

This is the portable executable header, containing 8 section definitions (.text, .data, .rdata, .eh_fram, .bss, .idata, .CRT, .tls).
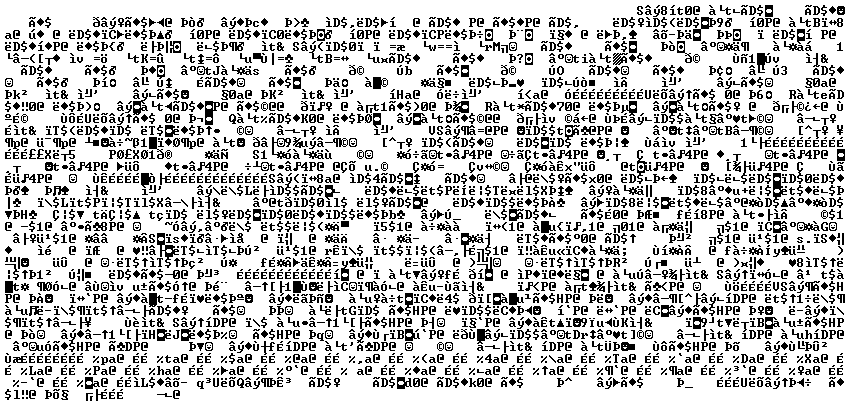
The code section is nearly as twice as huge as the one generated by Visual Studio compiler and of cause way more than we want inside our exe for calling 2 API functions.
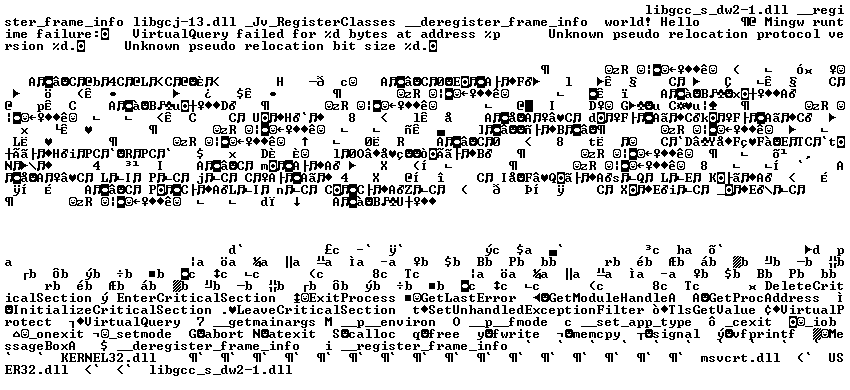
In the import section you can see that it not only includes the Microsoft Visual C Run-Time Library, but also the MingW runtime.
Let’s pimp the project to remove all the stuff that we did not want included in the exe file:
- Open the .pro file of your project and replace it with following:
CONFIG -= qt
DEFINES -= QT_LARGEFILE_SUPPORT UNICODE
SOURCES += main.c
QMAKE_CFLAGS += -fno-asynchronous-unwind-tables
QMAKE_LFLAGS += -static-libgcc -nostdlib
LIBS = -lkernel32 -lmsvcrt -luser32
- If you try to build the project now you will get an linker error about undefined reference to `__main’. To fix this issue we just rename the main function in our c file:
#include <windows.h>
void __main()
{
MessageBoxA(0, "Hello", "world!", MB_OK);
ExitProcess(0);
}
Recompile the project and your compiled exe will have a size of 2.048 byte and look so fresh and so clean like this:
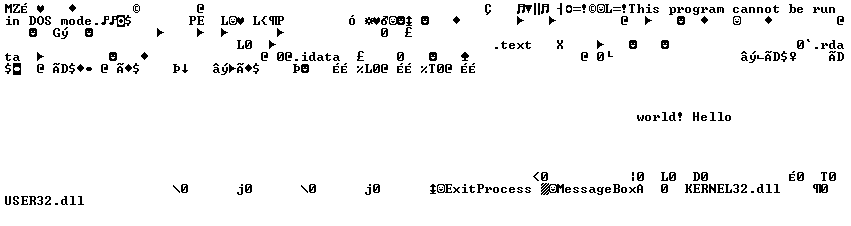